说明
因为一些原因,我们提供给客户的sdk,只能是jar包形式的,一些情况下,sdk里面有native库的时候,就不太方便操作了,此篇文章主要解决如何把so库放入jar包里面,如何打包成jar,以及如何加载。
1.如何把so库放入jar包
so库放入jar参考此文章ANDROID将SO库封装到JAR包中并加载其中的SO库
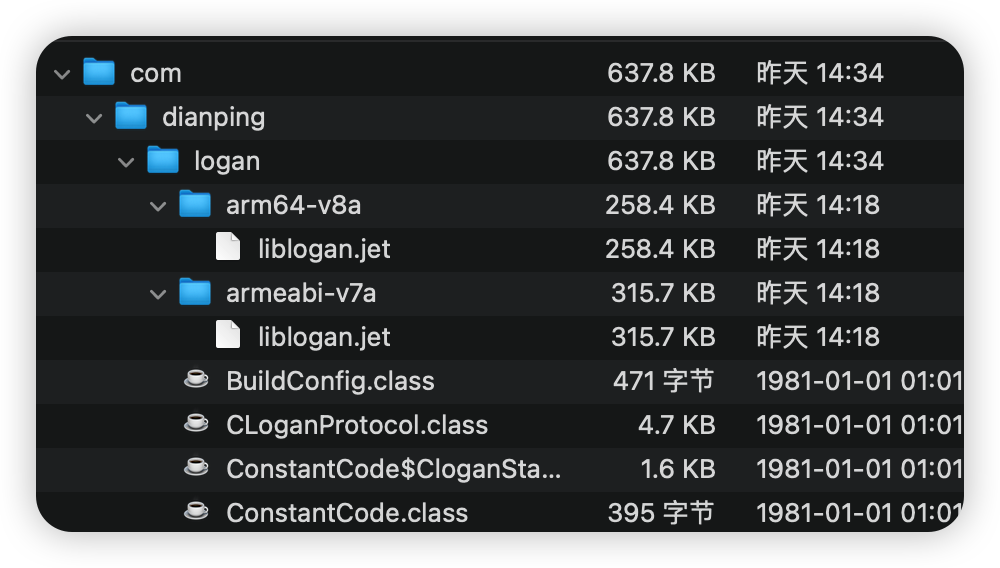
将so库改成.jet后缀,放置和加载so库的SoLoader类同一个目录下面。
2.如何使用groovy打包jar
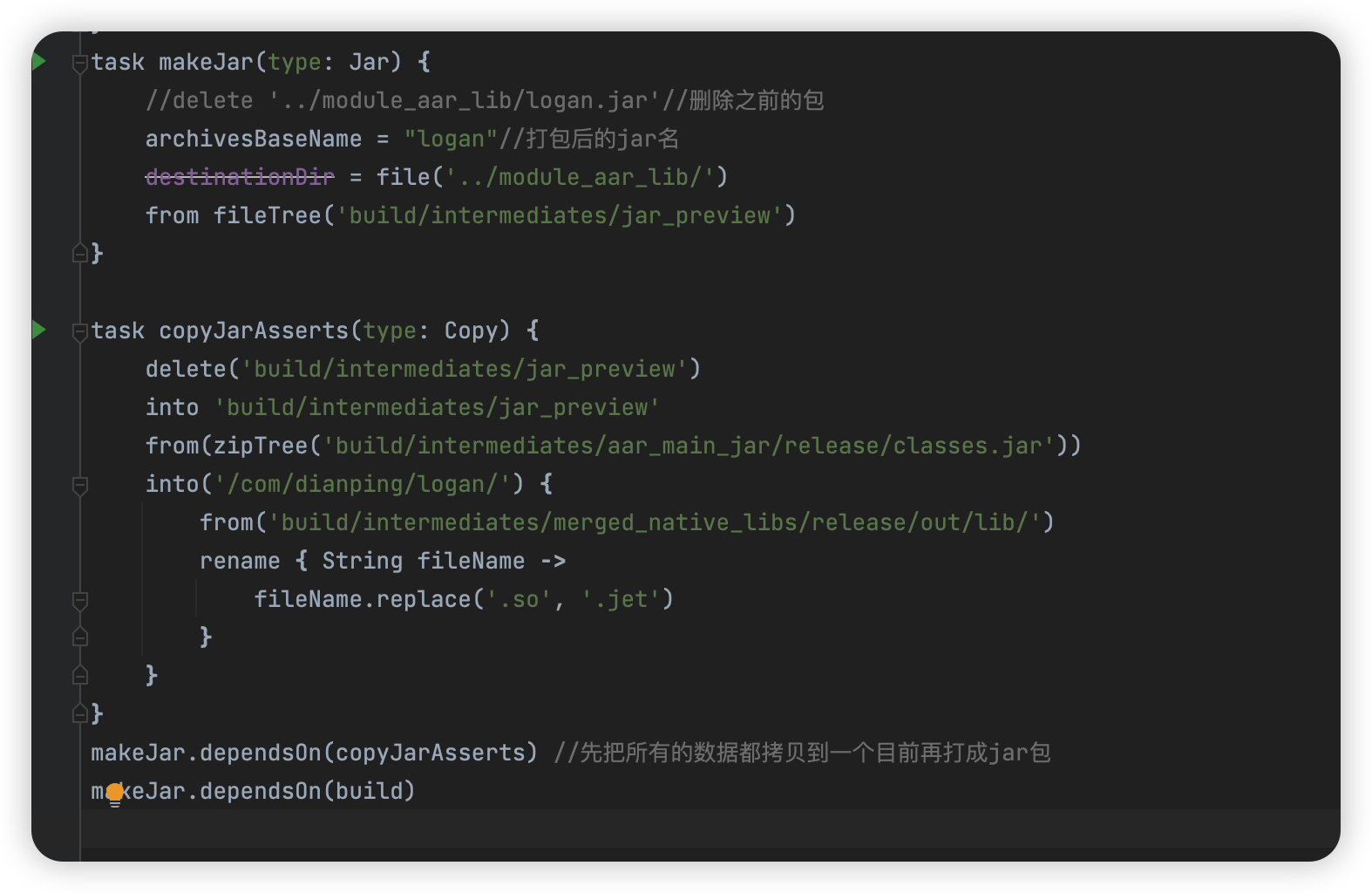
先把需要打包的class放置到同一个文件夹下面,然后打包即可,利用groovy的copy task完成这项工作非常简单。
3.如何加载jar包里面的so
3.1.首先判断当前jar里面是否存在so
1
| InputStream inputStream = SoLoader.class.getResourceAsStream("/com/dianping/logan/arm64-v8a/liblogan.jet");
|
如果inputStream不为空就表示存在。
3.2.拷贝
判断是否已经把so库拷贝到手机里面了,如果没有拷贝过就进行拷贝,这个代码逻辑很简单。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131
| public class SoLoader { private static final String TAG = "SoLoader";
public static String getPath() { String path = GlobalCtx.getApp().getFilesDir().getAbsolutePath(); return path; }
public static String get64SoFilePath() { String path = SoLoader.getPath(); String v8a = path + File.separator + "jniLibs" + File.separator + "arm64-v8a" + File.separator + "liblogan.so"; return v8a; }
public static String get32SoFilePath() { String path = SoLoader.getPath(); String v7a = path + File.separator + "jniLibs" + File.separator + "armeabi-v7a" + File.separator + "liblogan.so"; return v7a; }
public static boolean jarMode() { boolean jarMode = false; InputStream inputStream = SoLoader.class.getResourceAsStream("/com/dianping/logan/arm64-v8a/liblogan.jet"); if (inputStream != null) { jarMode = true; try { inputStream.close(); } catch (IOException e) { e.printStackTrace(); } } return jarMode; }
public static boolean alreadyCopySo() { String v8a = SoLoader.get64SoFilePath(); File file = new File(v8a); if (file.exists()) { String v7a = SoLoader.get32SoFilePath(); file = new File(v7a); return file.exists(); } return false; }
public static boolean copyLoganJni() { boolean load; File dir = new File(getPath(), "jniLibs"); if (!dir.exists()) { load = dir.mkdirs(); if (!load) { return false; } } File subdir = new File(dir, "arm64-v8a"); if (!subdir.exists()) { load = subdir.mkdirs(); if (!load) { return false; } } File dest = new File(subdir, "liblogan.so"); load = copySo("/com/dianping/logan/arm64-v8a/liblogan.jet", dest); if (load) { subdir = new File(dir, "armeabi-v7a"); if (!subdir.exists()) { load = subdir.mkdirs(); if (!load) { return false; } } dest = new File(subdir, "liblogan.so"); load = copySo("/com/dianping/logan/armeabi-v7a/liblogan.jet", dest); } return load; }
public static boolean copySo(String name, File dest) { InputStream inputStream = SoLoader.class.getResourceAsStream(name); if (inputStream == null) { Log.e(TAG, "inputStream == null"); return false; } boolean result = false; FileOutputStream outputStream = null; try { outputStream = new FileOutputStream(dest); int i; byte[] buf = new byte[1024 * 4]; while ((i = inputStream.read(buf)) != -1) { outputStream.write(buf, 0, i); } result = true; } catch (IOException e) { e.printStackTrace(); } finally { try { inputStream.close(); } catch (IOException e) { e.printStackTrace(); } if (outputStream != null) { try { outputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } return result; }
}
|
3.3.加载
首先判断当前应用是32位还是64位Process.is64Bit();
。然后加载对应的32或者64位的so。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| static { try { if (SoLoader.jarMode()) { if (SoLoader.alreadyCopySo()) { sIsCloganOk = loadLocalSo(); } else { boolean copyLoganJni = SoLoader.copyLoganJni(); if (copyLoganJni) { sIsCloganOk = loadLocalSo(); } } } else { System.loadLibrary(LIBRARY_NAME); sIsCloganOk = true; } } catch (Throwable e) { e.printStackTrace(); sIsCloganOk = false; } }
static boolean loadLocalSo() { boolean bit = Process.is64Bit(); if (bit) { String v8a = SoLoader.get64SoFilePath(); try { System.load(v8a); return true; } catch (Throwable e) { e.printStackTrace(); return false; } } else { String v7a = SoLoader.get32SoFilePath(); try { System.load(v7a); return true; } catch (Throwable e) { e.printStackTrace(); return false; } } }
|